Firebase Cloud Messaging (FCM) or push notification is a robust solution for sending real-time notifications to the web, iOS, and Android devices.
Integrating FCM with NestJS is straightforward, and after reading this article, we will be able to implement it seamlessly.
FCM push notifications are widely popular today to keep users informed about the latest developments, regardless of whether they are actively using the app.
I highly recommend checking out this video:
For the demo, I’ll use:
- Firebase Cloud messaging service
- NestJS backend server
- Swagger for API endpoints
- Environment variables for storing configurations
Requisite
The latest version of the node should be installed on your system. We can test it with this command. If Node is installed on your system,
node -v
this command will return a version number like v22.1.0. The version may vary depending on the Node installed on your system.
Implementation
Setup NestJs project
Our first step would be setting up the NestJS project. Since we have already confirmed that Node is installed on our system, we can proceed to install the NestJS global CLI using this command
npm i -g @nestjs/cli
Here is the command to create a new NestJS project. For now, we will create a push-notification project using this command
nest new push-notification
Install dependencies
Now we will install the dependencies required for this project:
- @nestjs/config for adding and using the .env file
- @nestjs/swagger for adding API endpoint documentation
- firebase-admin for the Firebase SDK, which will enable us to use the Firebase Cloud Messaging Service
npm install --save @nestjs/config @nestjs/swagger firebase-admin
Create notification resource
Let’s create a NestJS resource named notification. This command will create a notification module with a controller, service, entity, and DTO. We will use this to create an endpoint for sending notifications to a device.
nest g resource notification --no-spec
Integrate Swagger(optional)
You can skip this part. We will integrate Swagger into our project to see the endpoints of the above resource. I am using the app name ‘Push Notification,’ but you can replace it with your own. Copy this code into main.ts
as shown in the image below.
const config = new DocumentBuilder()
.setTitle('Push Notification')
.setDescription(
'The API details of the business solution for the Push Notification Demo Application.',
)
.setVersion('1.0')
.addTag('Notification')
.addBearerAuth()
.build();
const document = SwaggerModule.createDocument(app, config);
SwaggerModule.setup('api', app, document);
Don’t forget to import Swagger dependencies as shown in the image.

Let’s start the project in development mode with this command.
npm run start:dev
When the project runs, we can check whether the Swagger is integrated successfully on http://localhost:3000/api as shown in the images.

Integrate .env (optional)
it’s always good practice to keep configuration settings in an environment file. For this purpose, we have already installed an npm package.
Create the .env file in the root directory, not in the src directory, and paste the code below into your app.js.For now, we will only include the port. Later, we will add the FCM configuration as well.
PORT=3000
Update the AppModule by adding ConfigModule in the imports array like this:
imports[ConfigModule.forRoot(), NotificationModule]
Update main.ts to read values from .env
await app.listen(process.env.PORT);
Setup Firebase Project
It’s a really important step. Open the Firebase console in your Google account and create a project. Add an app according to your needs, such as Android, iOS, or web. Generate your Firebase private key, as we will use this in our NestJS backend.
If there is any issue, you can take help from the above video.

Generate a private key and update the .env file as shown below with dummy credentials for the Firebase project.
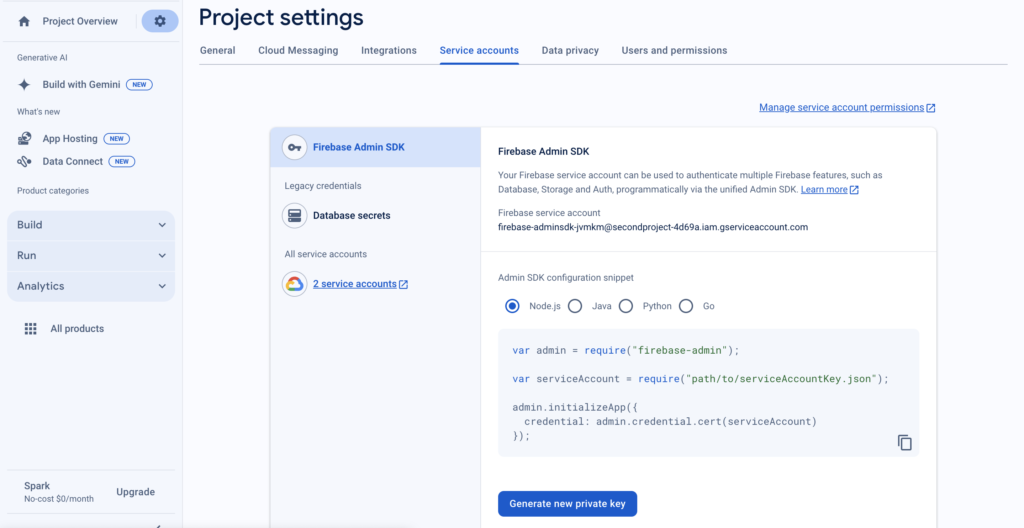
PROJECT_ID=zxcnmm
PRIVATE_KEY=-----BEGIN PRIVATE KEY-----\n
MIICdgIBADANBgkqhkiG9w0BAQEFAASCAmAwggJcAgEAAoGBAJGtnoAnAdHABIb1
sdfbsdfds+wf
-----END PRIVATE KEY-----\n
[email protected]
Note:If you paste the exact credentials, it will cause the error: ‘Invalid PEM formatted message.‘ at the new ServiceAccount. So, replace these values with those from the Firebase file.
Discover more excellent articles from The CodeMoode
- Understanding the JavaScript Double Exclamation Marks (!!)
- Are you worried about whether Devin AI will take your job?
- Docker Image vs. Container: A Detailed Article Explaining Their Differences
- Docker vs. Kubernetes: A Comprehensive Comparison
- Unlocking the Power of Promise.allSettled() in TypeScript
Setup firebase in NestJs project
open the notification module and then add firebase-admin.provider.ts along with a service file and paste the code.
This code sets up Firebase Admin in a NestJS application by importing the Firebase Admin SDK, creating a provider, and initializing the Firebase app with credentials from environment variables. The provider is then exported for use throughout the application.
import * as admin from 'firebase-admin';
export const firebaseAdminProvider = {
provide: 'FIREBASE_ADMIN',
useFactory: () => {
const defaultApp = admin.initializeApp({
credential: admin.credential.cert({
projectId: process.env.PROJECT_ID,
clientEmail: process.env.CLIENT_EMAIL,
privateKey: process.env.PRIVATE_KEY?.replace(/\\n/g, '\n'),
}),
});
return { defaultApp };
},
};
update notification module and this file into service providers
providers: [firebaseAdminProvider, NotificationService]
Update Notification Controller
Let’s create a controller endpoint to send a notification to an Android device (in our case). For this, first, create a notification DTO named send-notification.dto.ts as shown in the code. Here is some explanation: ‘Title’ would be the title of the notification, where ‘body’ will be the text of the subscription, and ‘device id’ will be obtained by Firebase SDK from the client device (this device is really important as it identifies each device) to send the notification to. ApiProperty is for Swagger documentation. A POST request is created with the ‘send notification’ type so we can send notifications.
here is send-notification.dto.ts
import { ApiProperty } from '@nestjs/swagger';
export class sendNotificationDTO {
@ApiProperty()
title: string;
@ApiProperty()
body: string;
@ApiProperty()
deviceId: string;
}
here is notification.controller.ts to send notification
@Post()
sendNotification(@Body() pushNotification: sendNotificationDTO) {
this.notificationService.sendPush(pushNotification);
}
Update Notification Service
Import Firebase dependencies so that we can utilize the notification service.
import * as firebase from 'firebase-admin';
Copy this code into your notification service here is the explanation of this code
- sendPush is an async function sending push notifications via Firebase.
- It constructs the payload from provided data like title and body.
- Specific configurations for Android and iOS platforms are set.
- Errors during the sending process are caught and logged.
async sendPush(notification: sendNotificationDTO) {
try {
await firebase
.messaging()
.send({
notification: {
title: notification.title,
body: notification.body,
},
token: notification.deviceId,
data: {},
android: {
priority: 'high',
notification: {
sound: 'default',
channelId: 'default',
},
},
apns: {
headers: {
'apns-priority': '10',
},
payload: {
aps: {
contentAvailable: true,
sound: 'default',
},
},
},
})
.catch((error: any) => {
console.error(error);
});
} catch (error) {
console.log(error);
return error;
}
}
Notification Received
When you hit this API endpoint to send a notification, providing a title, body, and device ID, you will receive a notification on the integrated app with the data you provided in that notification.

Conclusion
This article guides you through integrating Firebase Cloud Messaging (FCM) with NestJS for seamless push notification delivery.
It covers setting up NestJS, installing dependencies, configuring Firebase, and implementing notification endpoints and services.
By following these steps, you’ll efficiently notify users across web, iOS, and Android platforms, enhancing user engagement and experience.
In our exploration of handling website redirections, discover how to Implement Server-Side Redirects in Next.js for seamless navigation and optimization.
Leave a Reply